Object Instantiation Java Example

What Is An Abstract Class In Java Talksinfo
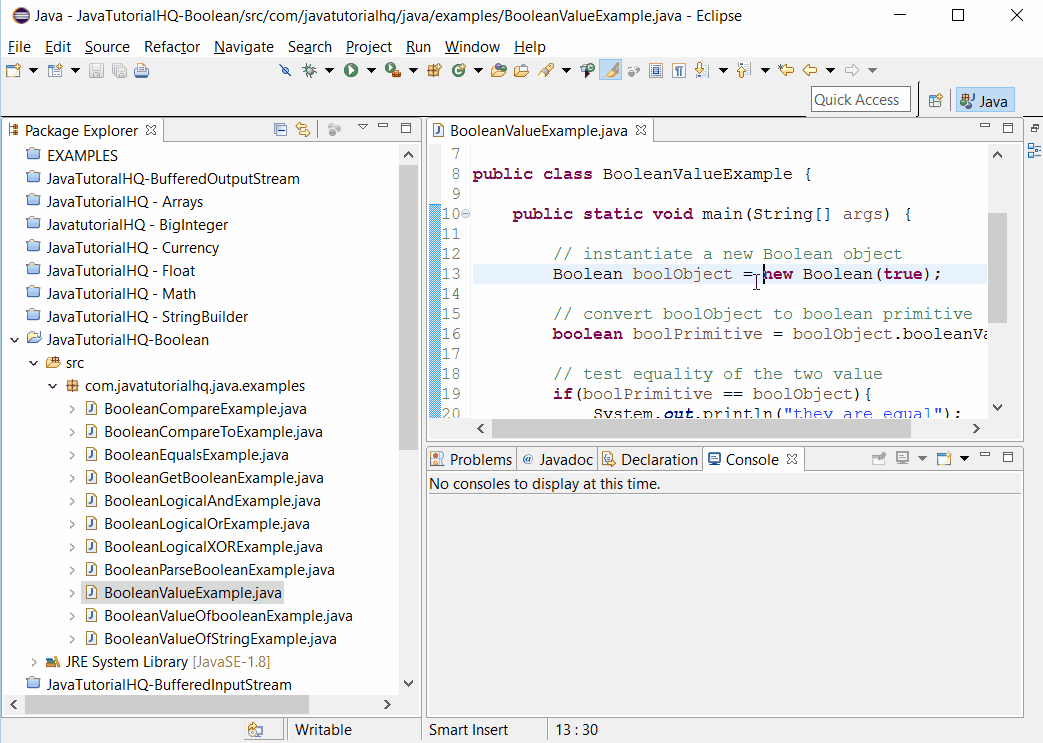
Q Tbn 3aand9gcqttfhvnjxgzwsaif3p1d6qezaawf4cdf4qtg Usqp Cau
Q Tbn 3aand9gctgygpyqeglidm9ymc74huqjchquzeft0vwg1y Isflfytrk1as Usqp Cau
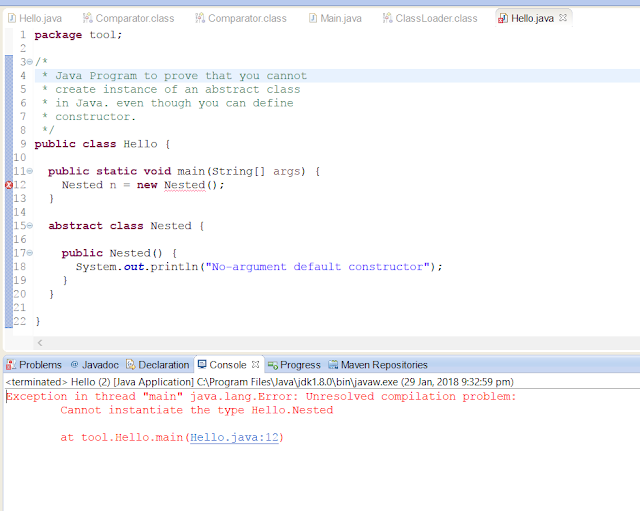
Is It Possible To Create Object Or Instance Of An Abstract Class In Java Java67

Introduction To Object Oriented Programming Java Programming

Java Array Of Arraylist Arraylist Of Array Journaldev
Each object decides what to do with a received message.

Object instantiation java example. Regardless of whether your run() method is in a Thread subclass or a Runnable implementation class, you still need a Thread object to do the work. The following are top voted examples for showing how to use java.lang.InstantiationException.These examples are extracted from open source projects. We do not need to implement the Cloneable interface and handle CloneNotSupportedException.;.
In Java, every class has its constructor that is invoked automatically when an object of the class is created. I guess you have enough knowledge of Object-oriented programming and Classes in Java. 1) In object-oriented programming, some writers say that you instantiate a class to create an object, a concrete instance of the class.
For Example, Pen is an object. Class DateApp { public static void main (String args) { Date today = new Date();. First, let us try to understand the what all a class contains in OOPS or in specific Java;.
When an object instance is created from a class, the class's constructor function is run to create it. In java linkedlist is a collection class that can be used both as a queue as well as a list. An object is a collection of properties, and a property is an association between a name (or key) and a value.
An instance variable is similar to a class variable. You don't have to append 'this' keyword explicitly unless there is an exceptional situation as in our example. Therefore, we need to typecast it to.
Java is an Object-Oriented Language. It falls under the category of the creational design pattern in Java. In addition to objects that are predefined in the browser, you can define your own objects.
Type in the following Java statements:. While object 2 is calling the set data method, an instance variable of object 2 is modified. Open your text editor and create a new file.
To instantiate is to create such an instance by, for example, defining one particular variation of object within a class, giving it a name, and locating it in some physical place. Instance Variable With Example In JAVA An instance variable is a variable defined in a class (i.e. This video continues from Tutorial 14.
In the following example, I create a Java interface named Animal, and then create a class named Dog which implements this interface. They can be marked transient;. This ID number is unique to each object and is therefore an instance variable.
It is actually an attribute of the class. The code set in bold are all variable declarations that associate a variable name with an object type.;. System.out.println(d instanceof Animal);//true } }.
In object-oriented programming, an object is an instance of a class. Posts about object instantiation written by noor. Every class you use or write inherits the instance methods of Object.You need not to use any of these methods, but, if you choose to do so, you may need to override them with code that is specific to your class.
Java is object-oriented programming language. Example of Instance Variable class Page { public String pageName;. The object you have instantiated is referred to as person.
In real life, a car is an object. An abstract class is extended by other classes and the methods should be implemented. Singleton class in Java.
} public static void main(String args) { Testing t = new Testing();. An Employee class. Every individual Object created from the class has its own copy of the instance method(s) of that class.
In Java, we can also use the clone method to create an object from an existing object. Declaring Objects (Also called instantiating a class). This chapter describes how to use objects.
JavaScript provides four different methods to create objects. The clone method returns a general Object reference. Every class is a descendant, direct or indirect, of the Object class.
Objects pass messages to each other. Doing this helps in avoiding ClassCastException at runtime. } } The main() method of the DateApp application creates a Date object named today.This single statement performs three actions:.
As a language that has the Object-Oriented feature, Java supports the following fundamental concepts − Polymorphism;. Java class instance – The following is the general syntax for creating an instance of a. Objects correspond to things found in the real world.
However, the copy constructor has some advantages over the clone method:. A property's value can be a function, in which case the property is known as a method. In all the previous GUI examples, we have been using the JOptionPane class to handle most of the responsibilities for displaying the dialog and the GUI items in the dialog like the title, the buttons, and the messages.Although JOptionPane is very useful and easy to use, it is rather restrictive, and you cannot rely only on JOptionPane for a.
Example of an object :. Java is an object-oriented programming language. This process of creating an object instance from a class is called instantiation — the object instance is instantiated from the class.
Java variables are two types either primitive types or reference types. For example, a graphics program may have objects such as “circle”, “square”, “menu”. From the above figure, the vehicle is an abstract class which is extended by SUV, Sedan, etc.
Java instanceof interface example. The instanceof operator's basic syntax is:. They’re stored in a single memory location, and they only “know” which object they belong to because the this pointer is passed when you call them.
In order to understand the instance vs object vs reference in Java.First, we need to understand What is Object and Memory Management in Java. From our Person class, we can now create some actual people:. Another example of java instanceof operator class Animal{} class Dog1 extends Animal{//Dog inherits Animal public static void main(String args){ Dog1 d=new Dog1();.
In this example, you can observe two classes namely Calculation and My_Calculation. > Object-oriented programming offers a sustainable way to write spaghetti code. Java object-oriented design – Java class instance.
// instance variable with private access } Rules for Instance variable in Java. If you have approach two (extending Thread class):. Factories, a commonly used design pattern in Java, have several advantages over direct object instantiation, such as hiding the details of instantiation, providing the ability to return a subtype of the factory's return type, and optionally returning an existing object rather than a new object.
The copy constructor is much easier to implement. The new keyword is a Java operator that creates the object. Creating Objects In Java, you create an object by creating an instance of a class or, in other words, instantiating a class.You will learn how to create a class later in Creating Classes.Until then, the examples contained herein create objects from classes that already exist in the Java environment.
Instance variables belong to an instance of a class. A Class is like an object constructor, or a "blueprint" for creating objects. For example, suppose Bicycle is a class then MountainBicycle, SportsBicycle, TouringBicycle, etc can be considered as objects of the class.
Following is an example demonstrating Java inheritance. In this tutorial, you'll learn about Java constructors, how to create and use them, and different types of constructors with the help of examples. As soon as you create this object using the new keyword, a new block of memory is created and object "abc" will now be pointing to that new block of memory, this is called as instantiation in java.
On the other hand, the garbage collector is a Java program that takes care of automatic memory management by deleting objects that are no longer reachable. Copy and paste the following program in a file with name My_Calculation.java. Bike abc = new Bike();.
Before casting an unknown object, the instanceof check should always be used. ― Paul Graham Fundamentals of object-oriented programming Object-oriented programming is a programming paradigm where everything is represented as an object. Instantiation is also known as an instance.
An online shopping system might have objects such as “shopping cart”, “customer”, and “product”. Declaring, Instantiating and Initializing an Object import java.util.Date;. The Object class, in the java.lang package sits at the top of the class hierarchy tree.
In Java programming, instantiating an object means to create an instance of a class. Or you could say that class attributes are variables within a class:. For example, if Dog extends Animal then object of Dog can be referred by either Dog or Animal class.
Regardless of which method you use, every method will provide the…. Java is an object-oriented programming language. Why to use new keyword in java to create an object Check out our website:.
A dog has states - color, name, breed as well as behaviors – wagging the tail, barking, eating. It lets you accrete programs as a series of patches. So, an object is the instance(result) of a class.
If a class has an instance variable, then a new instance variable is created and initialized to a default value as part of the object creation of class or subclass. For example, suppose you want to create a number of Bicycle objects and assign each a serial number, beginning with 1 for the first object. Creating an Object in Java.
In C++ and other similar languages, to instantiate a class is to create an object, whereas in Java, to instantiate a class creates a specific class. First, let us discuss how to declare a class, variables and methods then we will discuss access modifiers. Here is how we can create an object of a class.
Instance variables in Java are non-static variables which are defined in a class outside any method, constructor or a block. Remember, every thread of execution begins as an instance of class Thread. // instantiate a Testing class object int result = t.Sample(1);.
Therefore, now we are going to look at different ways to create objects in Java. Instance variables can use any of the four access levels;. Using extends keyword, the My_Calculation inherits the methods addition() and Subtraction() of Calculation class.
You can vote up the examples you like and your votes will be used in our system to generate more good examples. A class when declared using the keyword abstract is an abstract class in java. Java Class Instance Tutorial – Java Object Oriented Design Tutorials for beginners from Coding compiler.Here you will learn about Java class instance, null reference type, accessing the fields of a class, default initialization of fields.Learn Now.!.
Instance methods are not stored on a per-instance basis, even with virtual methods. Public class Testing{ private int Sample(int c) { int a = 1;. Color is white, known as its state.
Public class Round { // implementation details }. // use the instance t to invoke a method on it System.out.println(result);. In the previous chapter, we used the term "variable" for x in the example (as shown below).
Learn about the instanceof operator in Java. What is Abstract Class in Java?. You'll be going over the subsequent study points:.
A class consists of member variables (fields) and behavior (methods or functions) Student.java. A parameterized class cannot have objects instantiated from it unless it is first instantiated itself (hence the confusing name for this type of relationship). An object is an instance of a class.
Singleton class in Java is one of the Gangs of Four Design Patterns. - Definition & Example. Each of these statements has three parts (discussed in detail below):.
The first line creates an object of the Point class, and the second and third lines each create an object of the Rectangle class. It is used to write, so writing is its behavior. For more on object-oriented programming, consider the lesson entitled What is Instantiation in Java?.
An instance variable belongs to a class. To get a newly-created object off to a good start, its newly-allocated memory must be initialized to a proper initial state. Here’s the source code for this Java instanceof interface example:.
Any new employee object. Int b = 2;. Think of the common example that is the Employee class;.
All objects in Java are stored in our program's heap memory. A member variable) in which each instantiated object of the class has a separate copy, or instance. C = a + b;.
Introduction:in this blog we will learn about linkedlist.in general terms, linkedlist is a data structure where each element consist of three parts. An object is called an instance of a class. Here, I instantiate objects within the main class, calling the Account class.
What is instance variable in Java?. I'll then test a dog object (which is an instance of the Dog class) against the Animal interface. Java classes consist of variables and methods (also known as instance members).
Declaration, instantiation, and initialization. This process is taken care by the compiler itself. Instantiation would be simple.
An object is a real-world entity. Instantiation patterns are ways to create something in JavaScript. First part represents the link to the previous element, second part represents the value of the element and last one represents the next element.
To instantiate an object in Java, follow these seven steps. Instance of a class. They can be marked final;.
Its name is Reynolds;. In fact, the heap represents a large pool of unused memory, allocated for our Java application. The results in both languages are the same (executable files) so there is no difference in use, just in terminology.
The car has attributes, such as weight and color, and methods, such as drive and brake. Everything in Java is associated with classes and objects, along with its attributes and methods. In this chapter, we will look into the concepts - Classes and Objects.
(object) instanceof (type) Let's see a basic example for the instanceof operator.First, let's create a class Round:. A class is a template or blueprint from which objects are created. An object is a chunk of memory bundled with the code that manipulates memory.In the memory, the object maintains its state (the values of its instance variables), which can change and evolve throughout its lifetime.
Each instantiated object of the class has a separate copy or instance of that variable. Object − Objects have states and behaviors. // instance variable with public access private int pageNumber;.
A Singleton class in Java allows only one instance to be created and provides global access to all other classes through this single object or instance. When you create an instance of a class or simply called as an object for ex:.
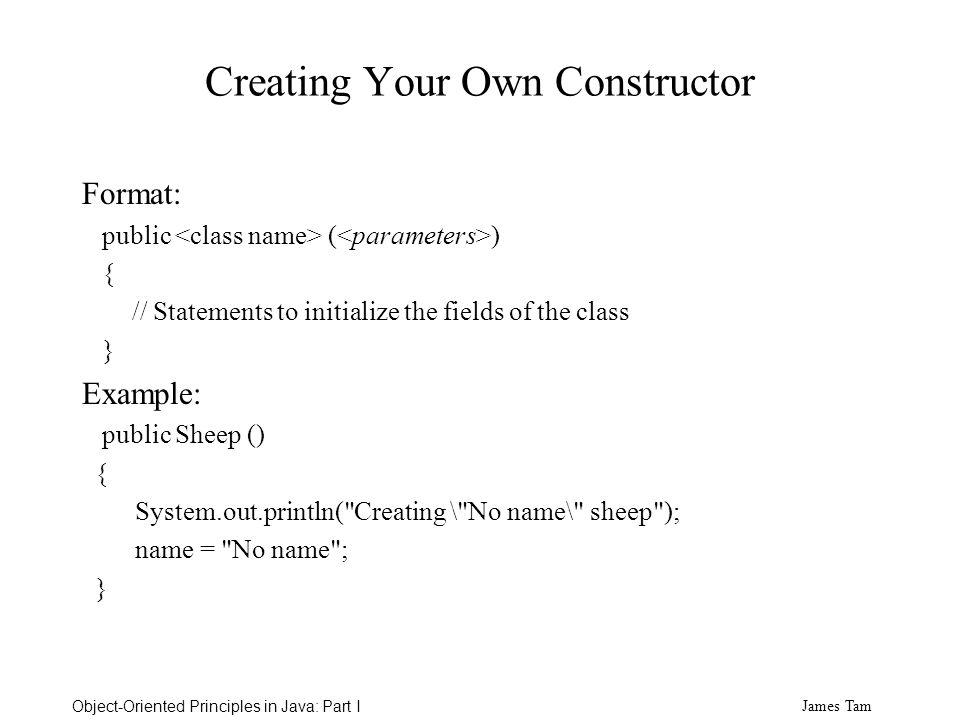
James Tam Object Oriented Principles In Java Part I Encapsulation Information Hiding Implementation Hiding Creating Objects In Java Class Attributes Ppt Download
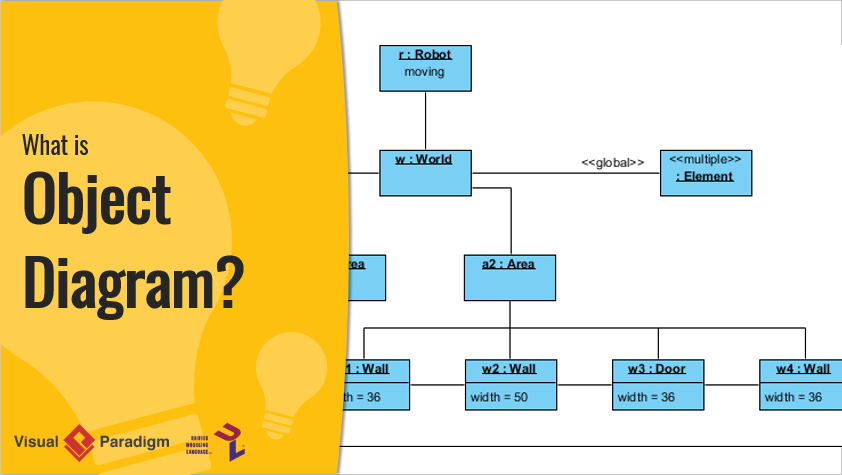
What Is Object Diagram
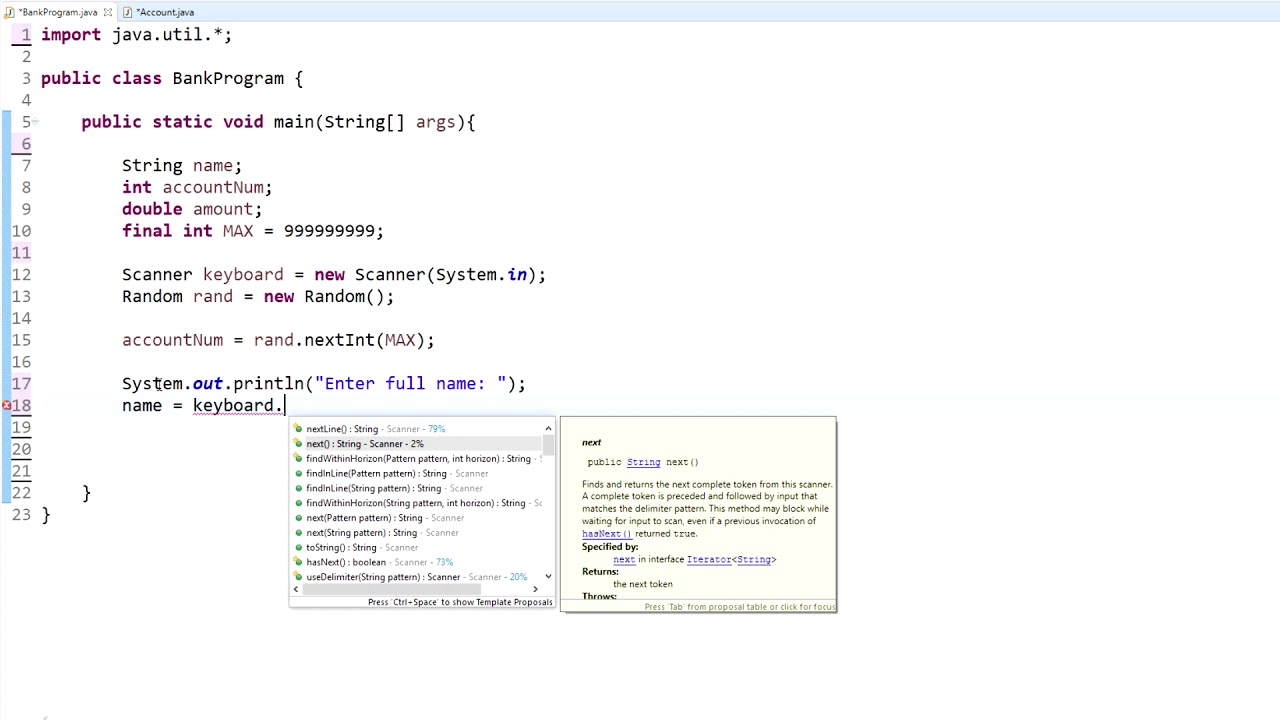
Java Programming Tutorial 18 Getting User Input And Creating Objects Youtube

What Is Instantiation In Java Definition Example Video Lesson Transcript Study Com
3

The Complete Guide To Javascript Classes
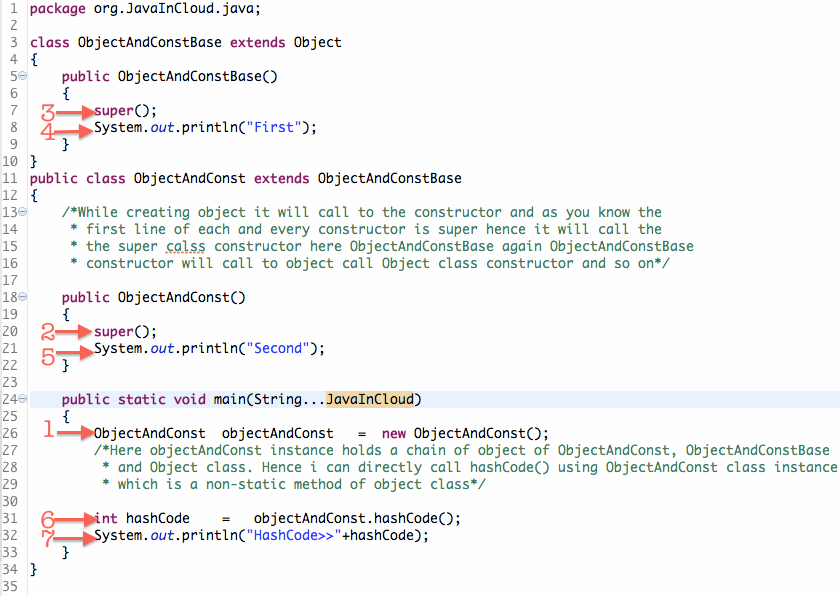
All About Object In Java Dzone Java
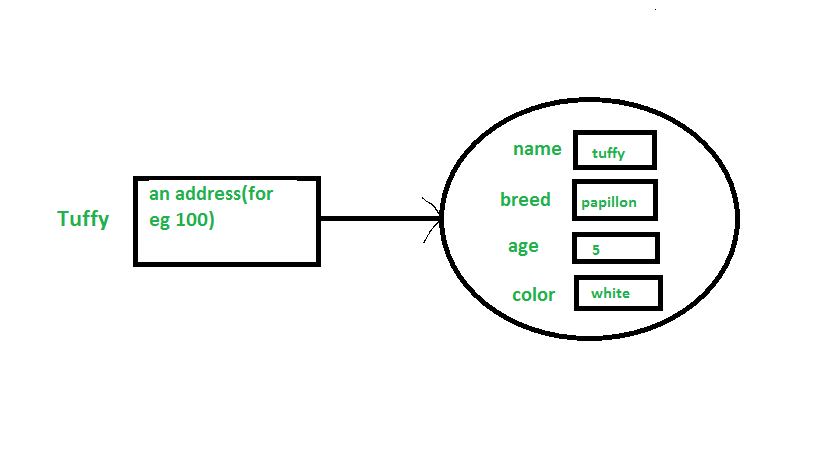
Classes And Objects In Java Geeksforgeeks
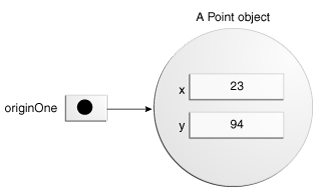
Creating Objects The Java Tutorials Learning The Java Language Classes And Objects
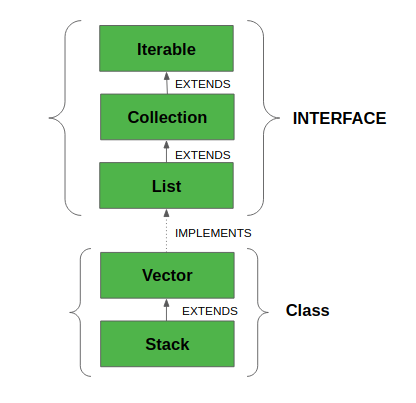
Stack Class In Java Geeksforgeeks
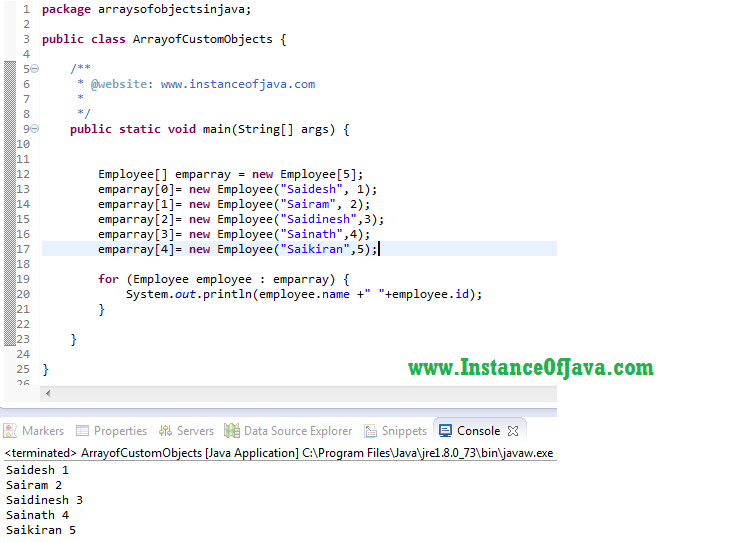
Creating Array Of Objects In Java Example Program Instanceofjava
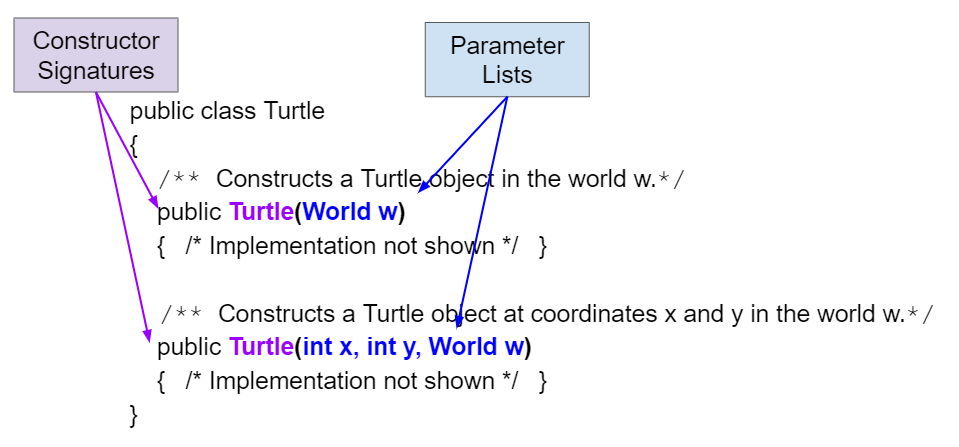
2 2 Creating And Initializing Objects Constructors Ap Csawesome
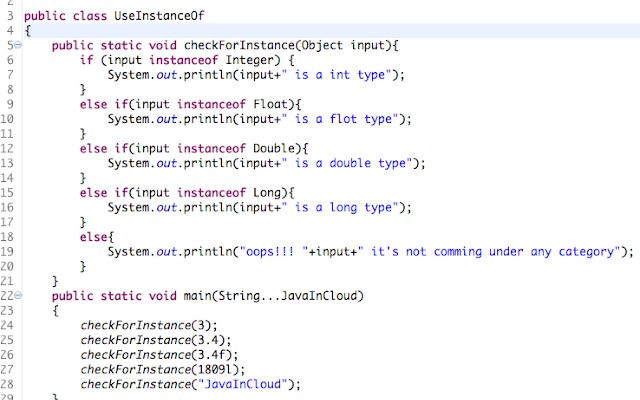
All About Object In Java Dzone Java
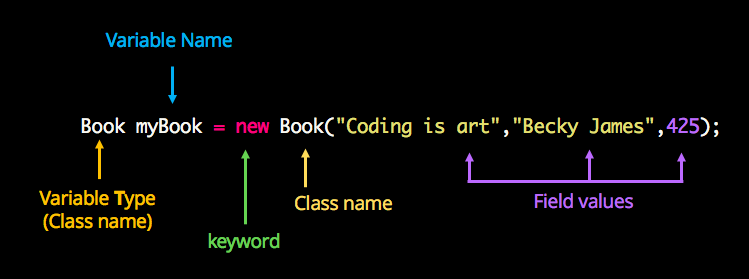
Define Objects And Their Attributes With Classes Learn Programming With Java Openclassrooms
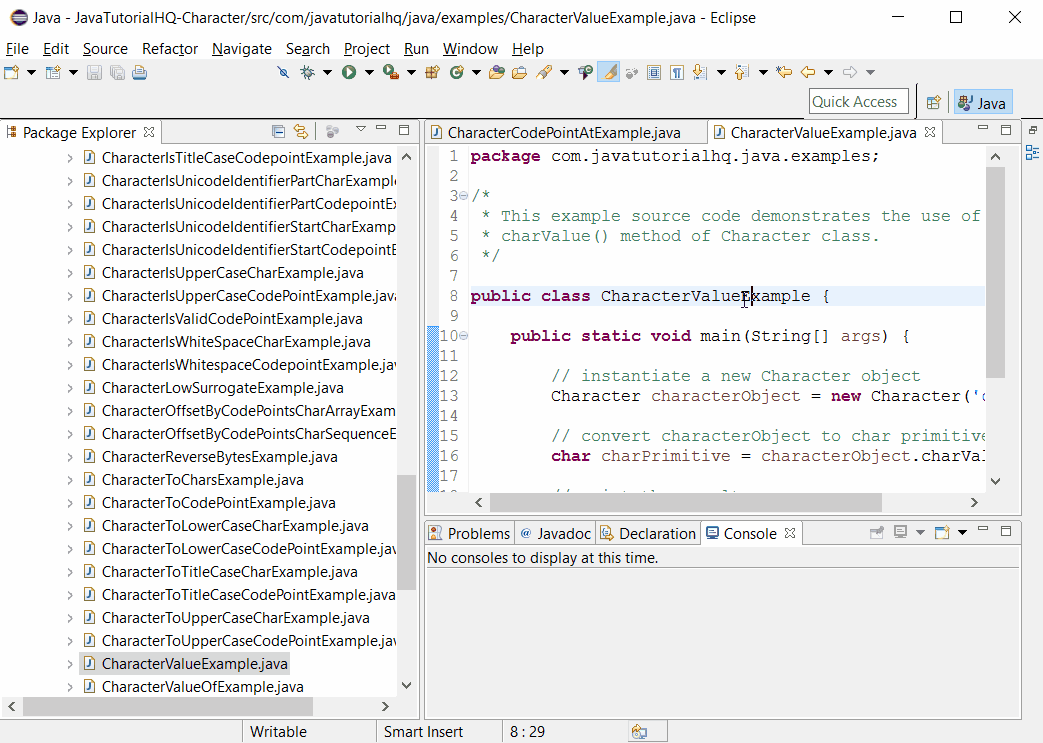
Q Tbn 3aand9gcrws3ohqfgc4kecey13n01dodhauimmqfzuyw Usqp Cau
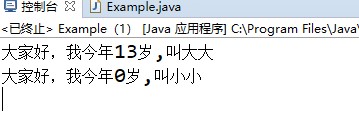
Java Basic Knowledge Object Oriented

How To Instantiate An Object In Java Webucator

Chapter 9 Introduction To Arrays Ppt Video Online Download

How Do I Instantiate An Object Of A Class Via Its String Name Web Tutorials Avajava Com
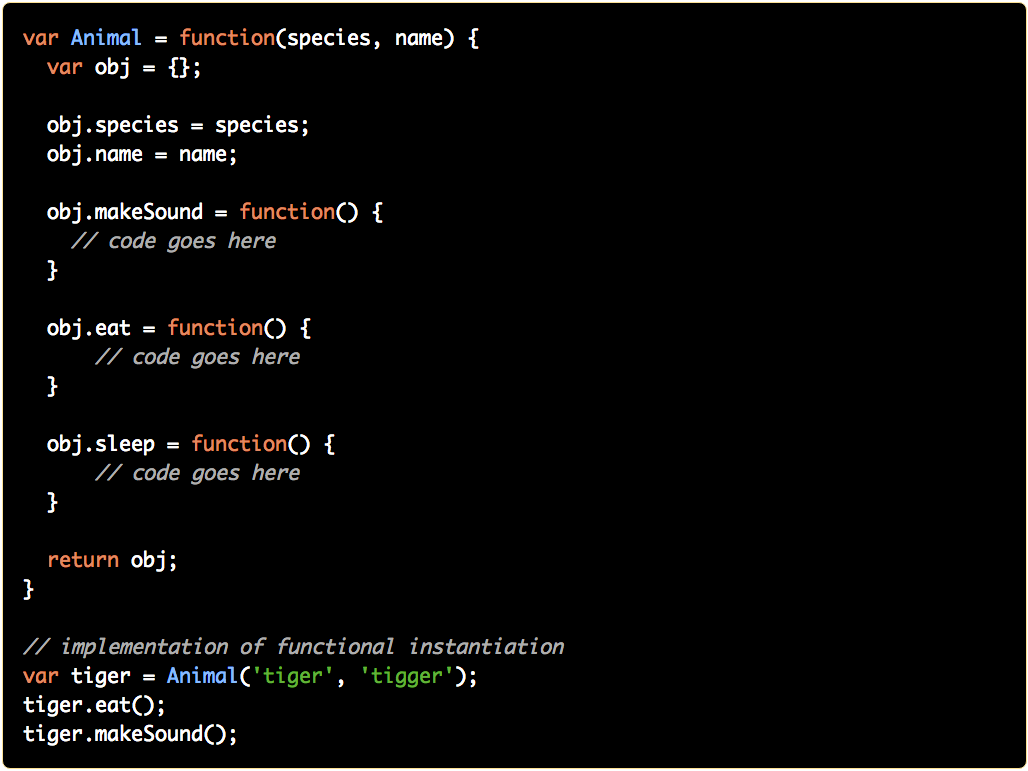
Instantiation Patterns In Javascript By Jennifer Bland Dailyjs Medium
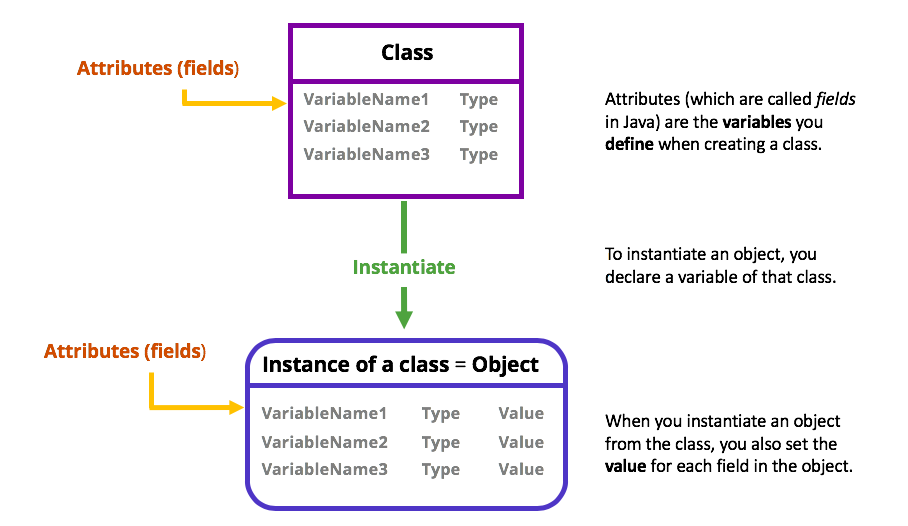
Define Objects And Their Attributes With Classes Learn Programming With Java Openclassrooms

Shortcut To Instantiate An Object In Visual Studio Stack Overflow
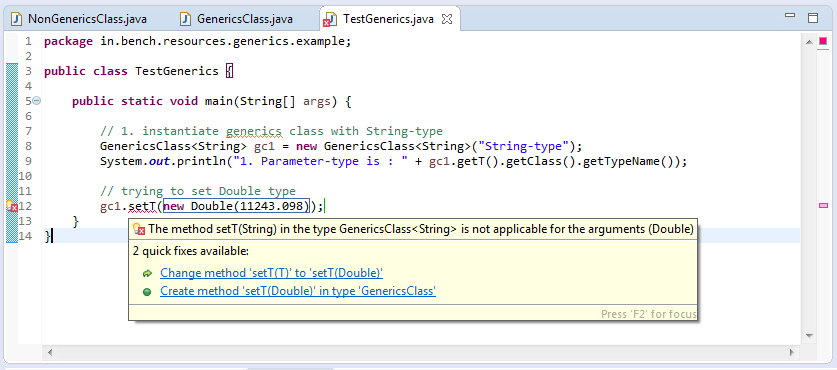
Generics Classes In Java Benchresources Net
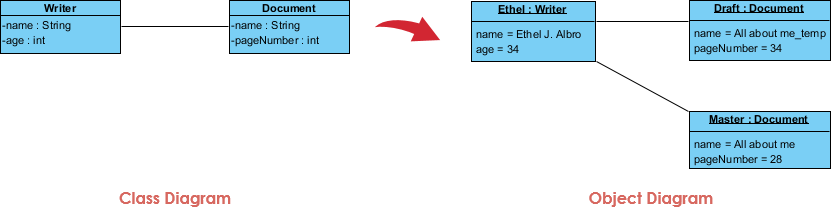
What Is Object Diagram

List Of Java Keywords Wikipedia
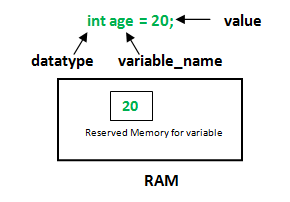
Variables In Java Geeksforgeeks

Hands On With Records In Java 14 A Deep Dive Jaxenter
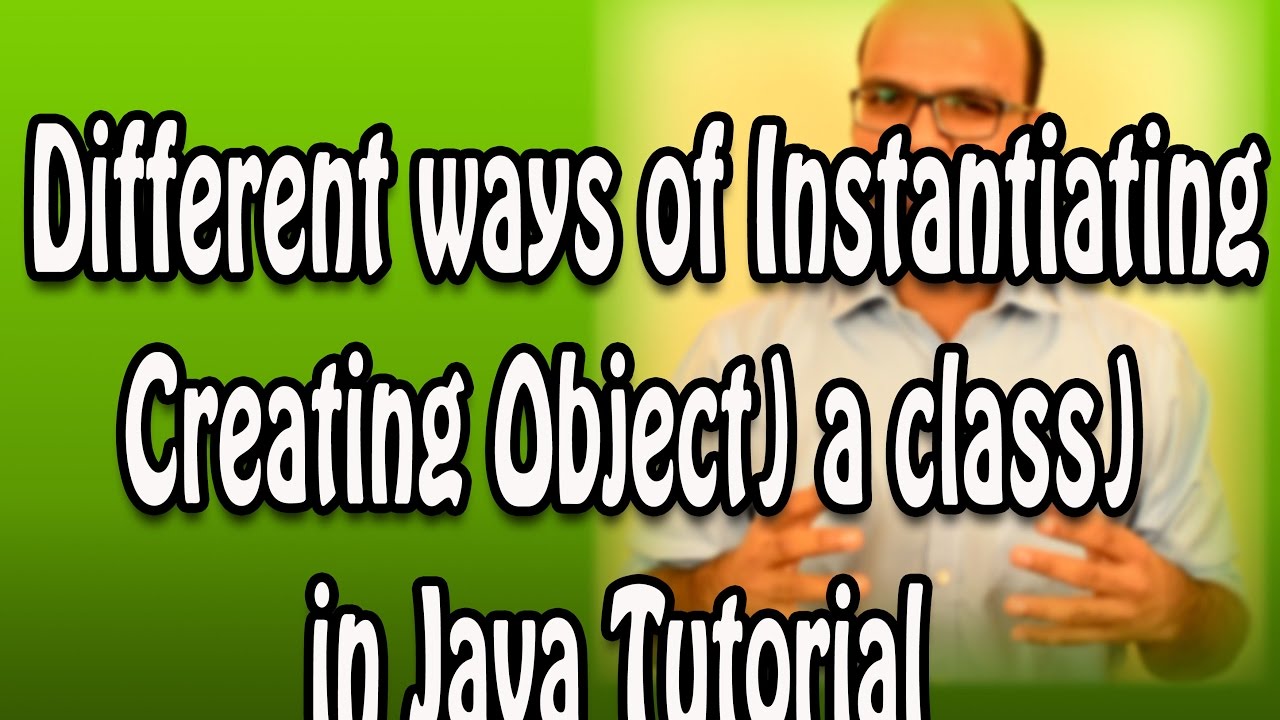
Different Ways Of Instantiating Creating Object A Class In Java Tutorial Youtube
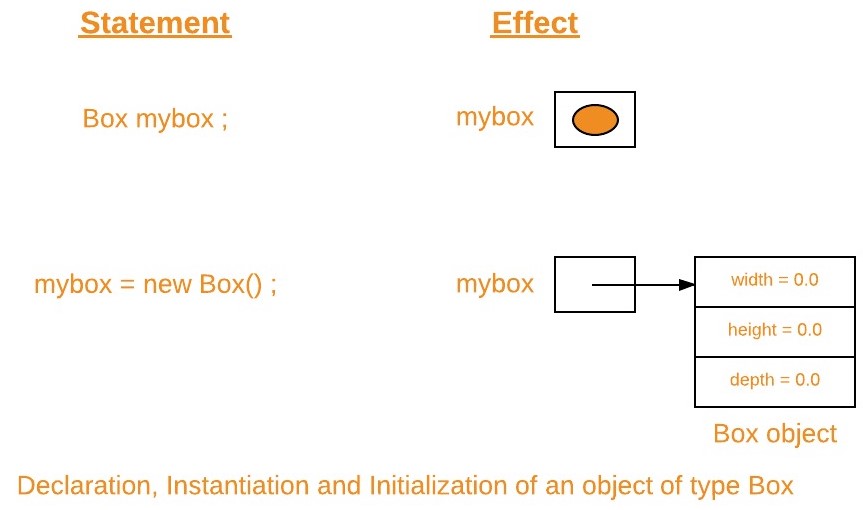
New Operator In Java Geeksforgeeks
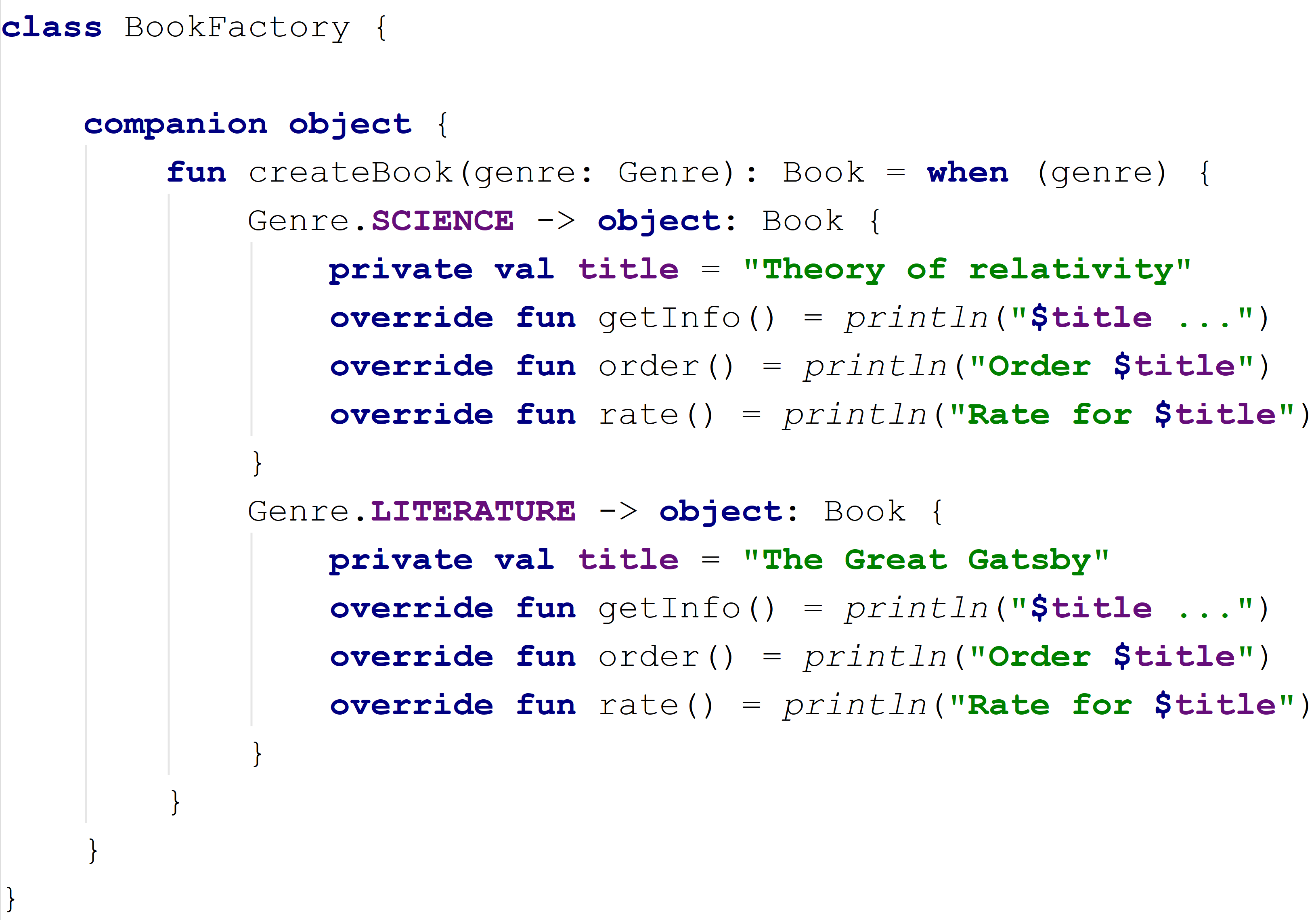
Factory Pattern In Kotlin Dzone Java
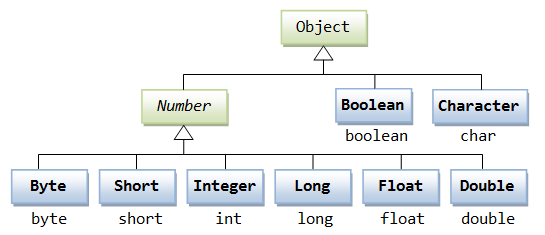
Java Programming Tutorial On Generics
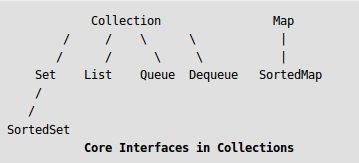
Initializing A List In Java Geeksforgeeks
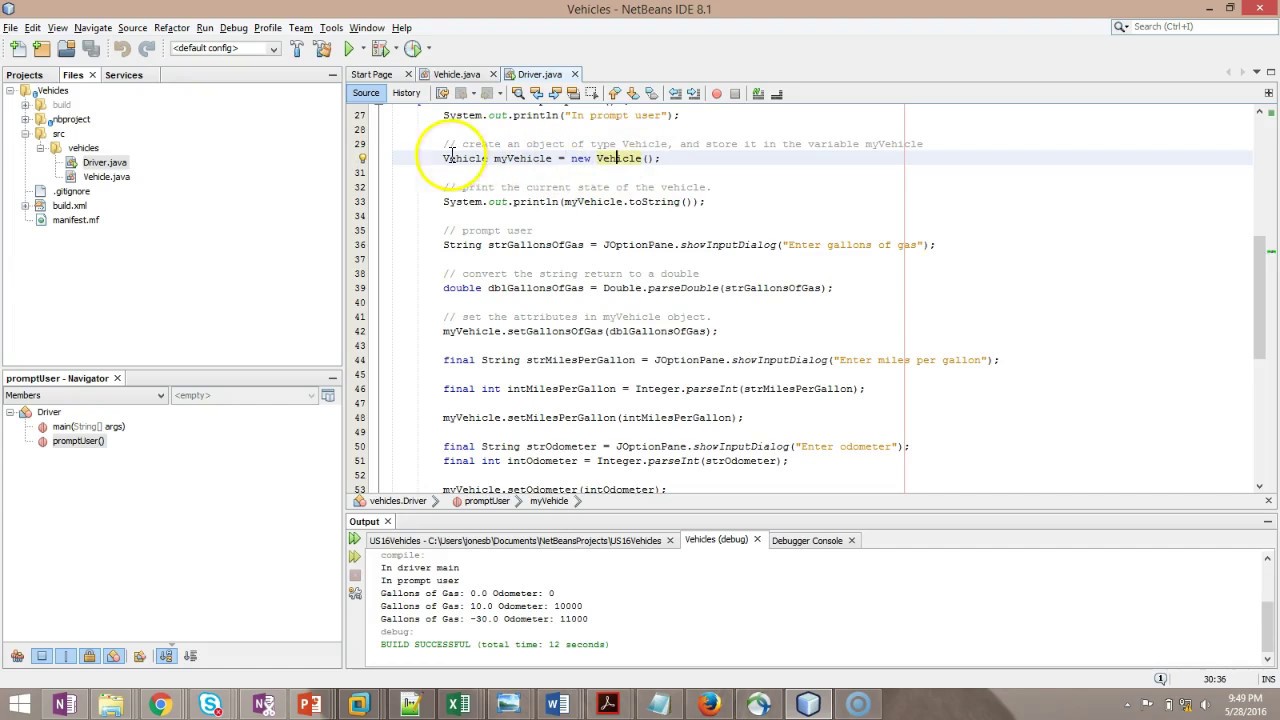
Creating Multiple Objects From One Class In Java Youtube

Hashmap Vs Concurrenthashmap Vs Synchronizedmap How A Hashmap Can Be Synchronized In Java Crunchify
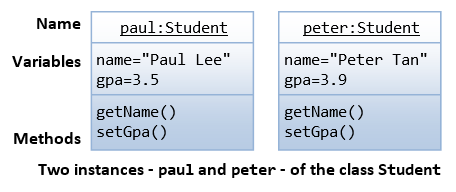
Oop Basics Java Programming Tutorial
How To Create An Immutable Class In Java With Example Programming Mitra
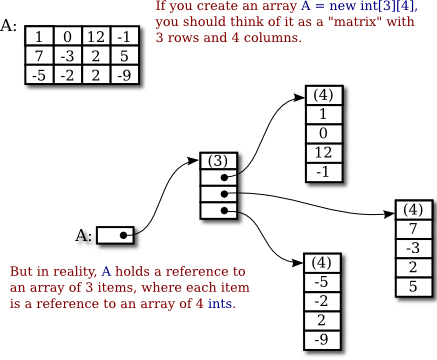
Javanotes 8 1 Section 7 5 Two Dimensional Arrays
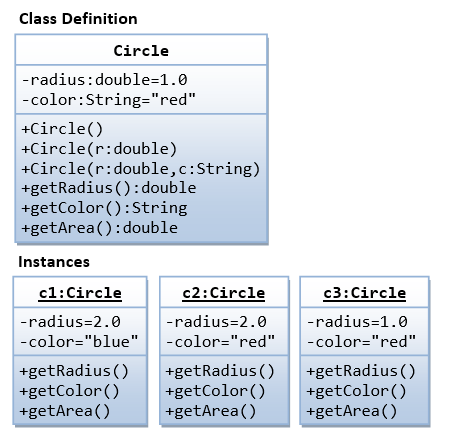
Oop Basics Java Programming Tutorial
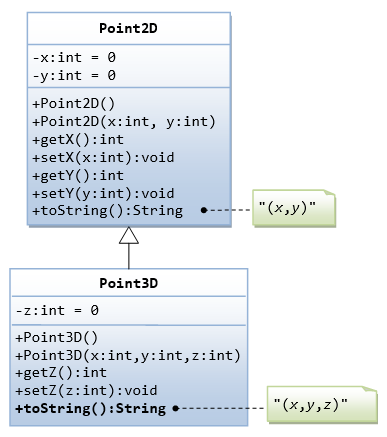
Oop Inheritance Polymorphism Java Programming Tutorial
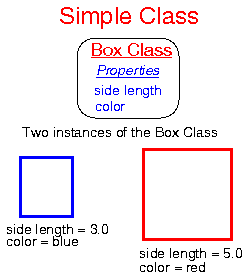
Understanding Classes And Objects
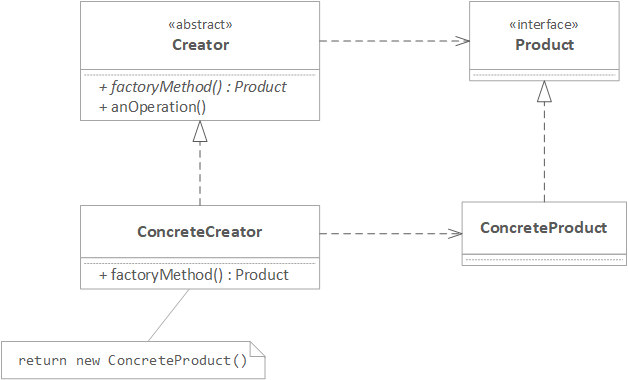
Java The Factory Method Pattern Dzone Java

Subclasses Superclasses And Inheritance
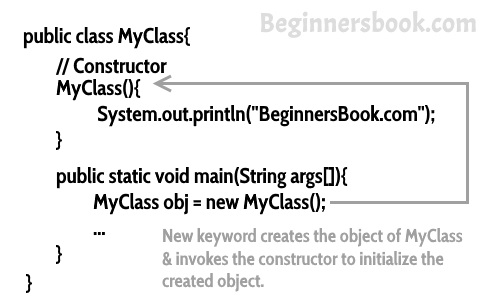
Constructors In Java A Complete Study
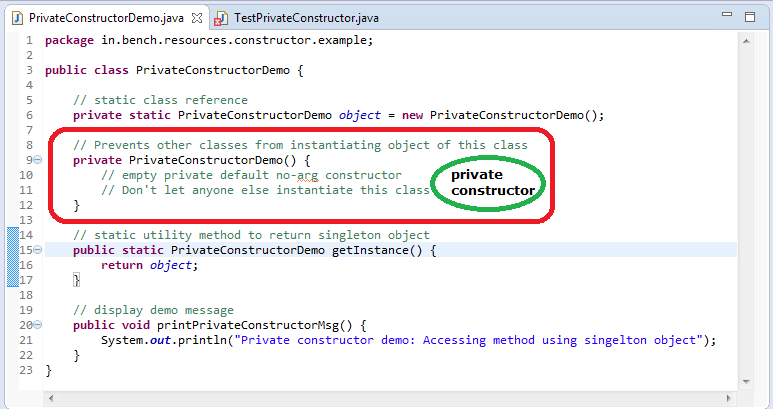
Java Private Constructor Benchresources Net
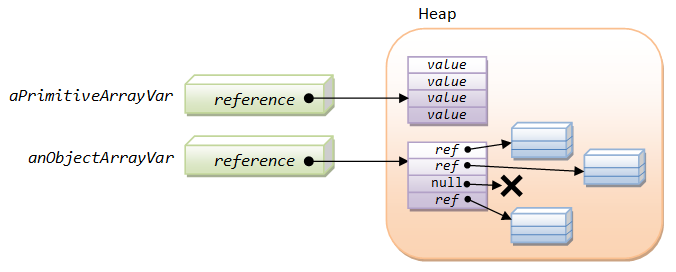
Oop Wrapping Up Java Programming Tutorial
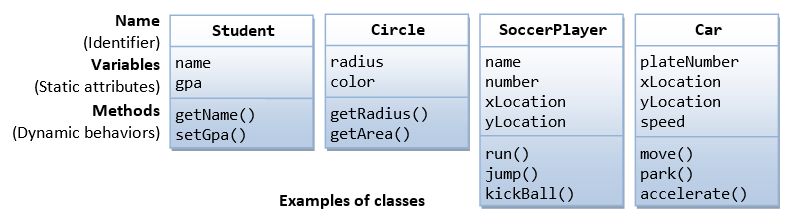
Oop Basics Java Programming Tutorial
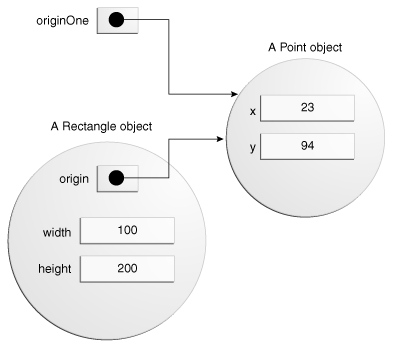
Creating Objects The Java Tutorials Learning The Java Language Classes And Objects
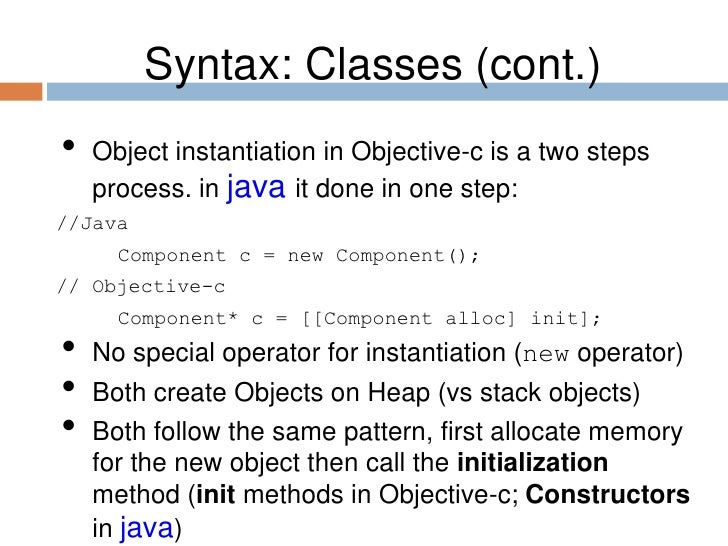
Objective C For Java Developers
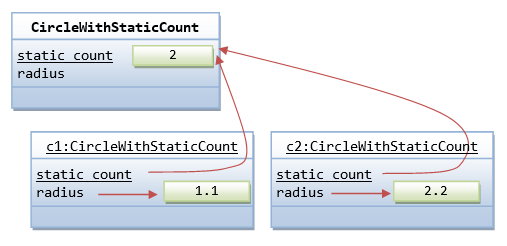
Oop Wrapping Up Java Programming Tutorial
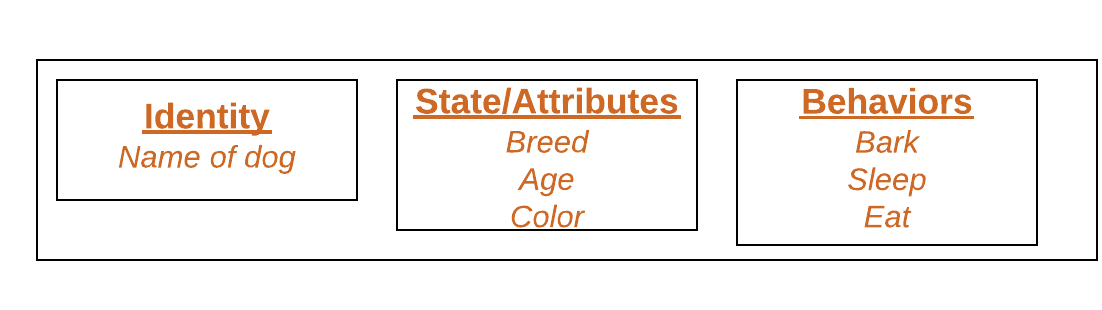
Classes And Objects In Java Geeksforgeeks

Instantiate Multiple Page Objects In 1 Test File Help Request Webtestit Discourse
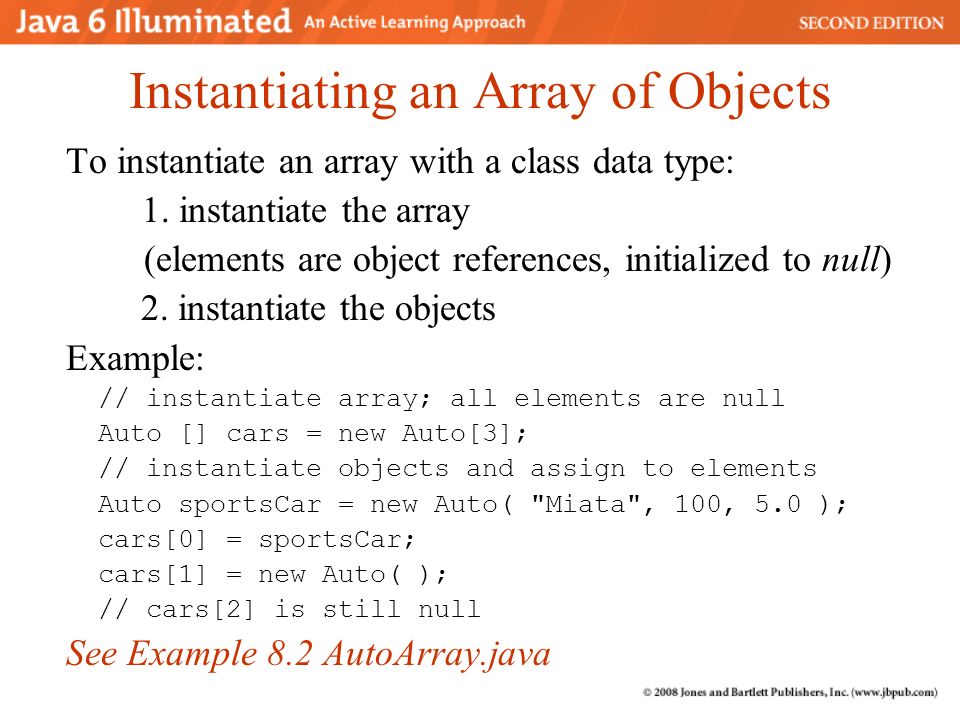
Chapter 8 Single Dimensional Arrays Topics Declaring And Instantiating Arrays Accessing Array Elements Writing Methods Aggregate Array Operations Using Ppt Download
Q Tbn 3aand9gctnyfnpuea9rxim2vvka3q3zb14xnm Mxo9qiaaz6voofexe7x8 Usqp Cau

How Do I Instantiate A Queue Object In Java Stack Overflow

Class Objects In Java Codebator

What Is A Class In Java Definition Examples Video Lesson Transcript Study Com

Do I Need Constructors To Create An Object In Java Quora

Java Hashmap Inline Initialization Java Tutorial Network
Q Tbn 3aand9gcrygyec3 V5f5smbjuszs4w1xi6yhhrj62taxlnbf5c Saqx8u6 Usqp Cau
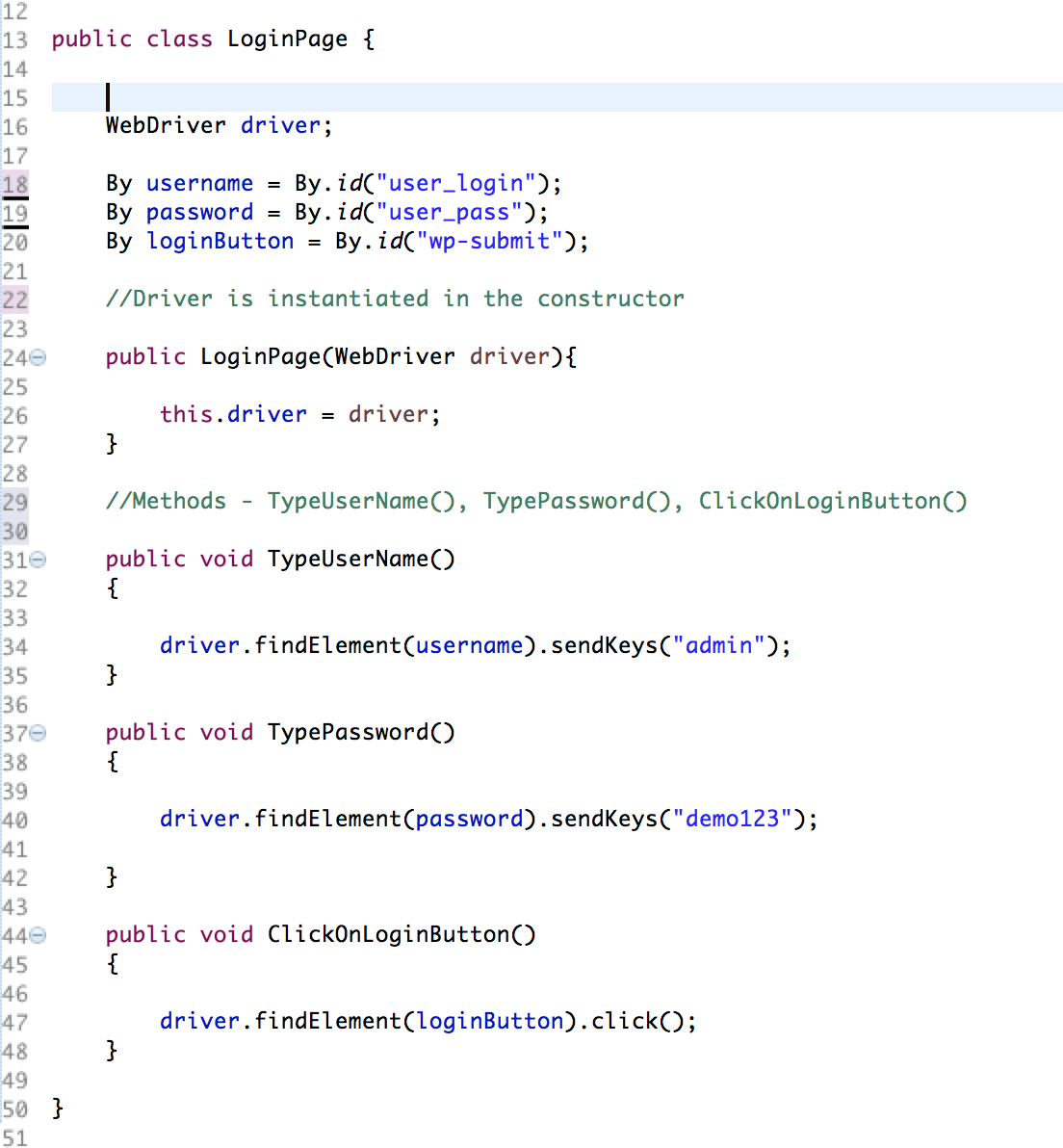
Page Object Model Using Selenium And Java By Sue Wild Medium
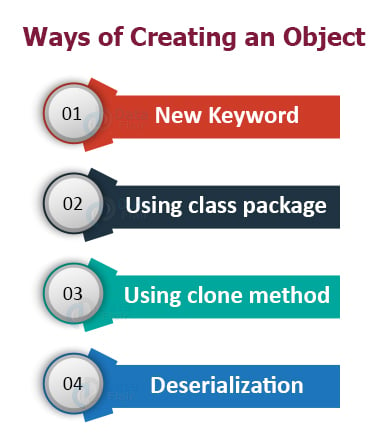
Classes And Objects In Java Fundamentals Of Oops Dataflair
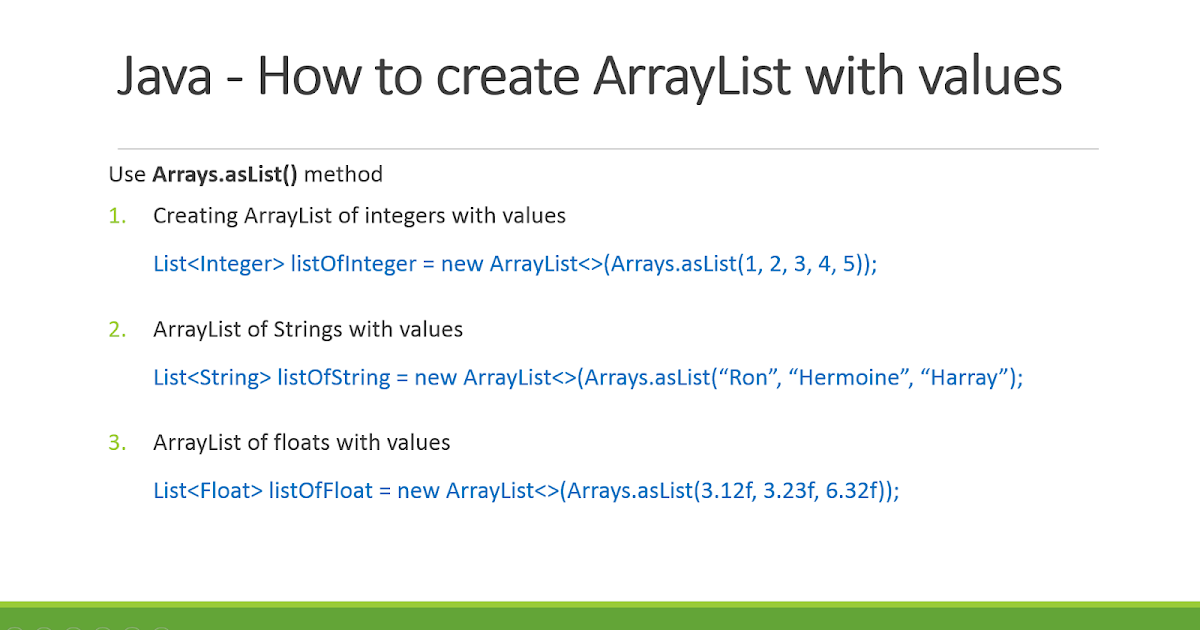
How To Declare Arraylist With Values In Java Examples Java67

How To Instantiate An Object In Java Webucator
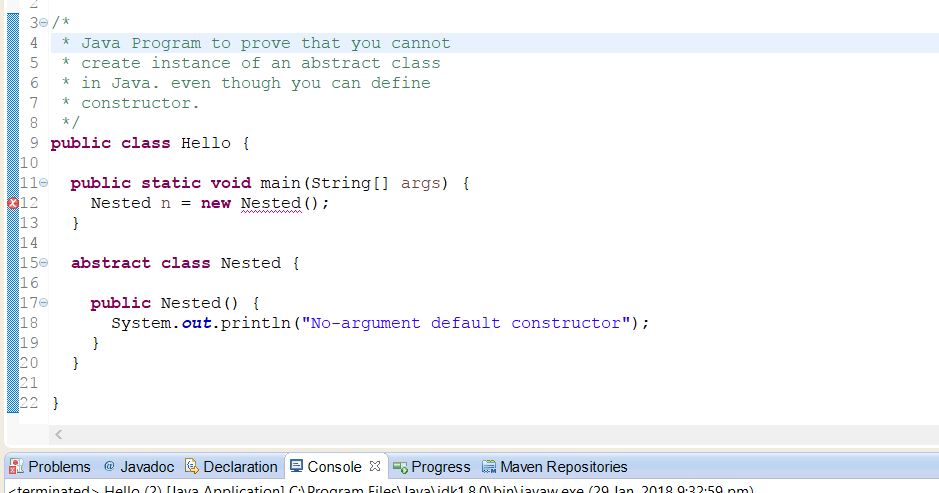
Is It Possible To Create Object Or Instance Of An Abstract Class In Java Java67

Object Oriented Javascript For Beginners Learn Web Development Mdn
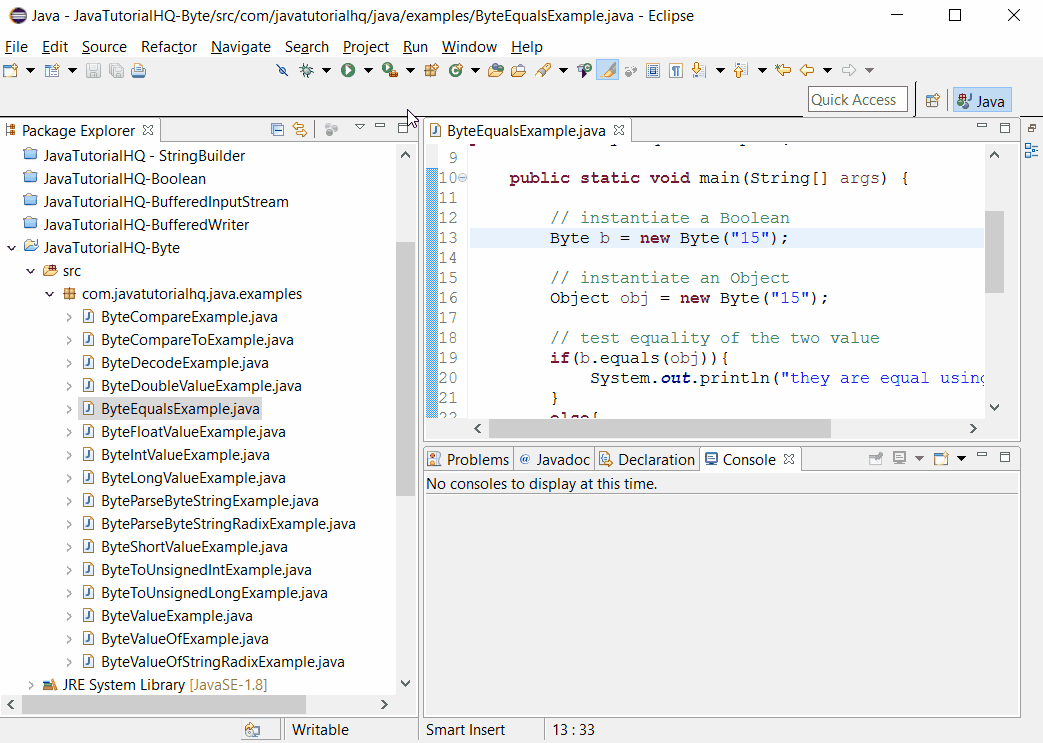
Q Tbn 3aand9gcqnebc8eqhcecmyeiyfysc5fhh0m337z1krxg Usqp Cau
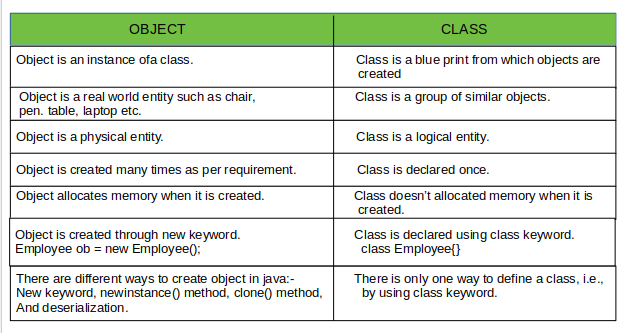
Understanding Classes And Objects In Java Geeksforgeeks
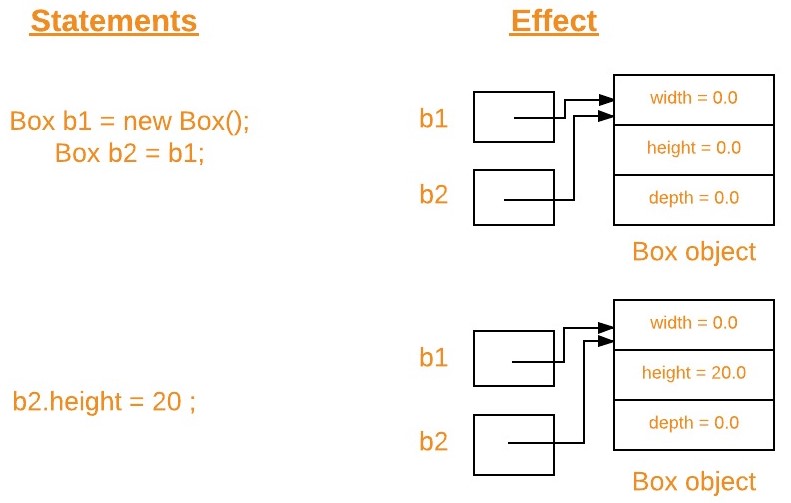
New Operator In Java Geeksforgeeks
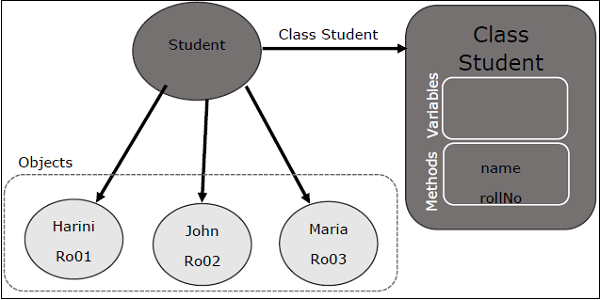
Scala Classes Objects Tutorialspoint
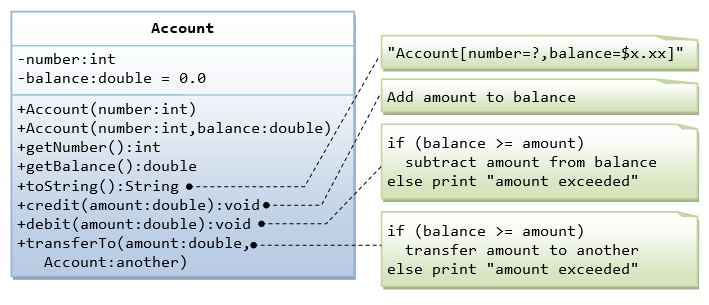
Oop Basics Java Programming Tutorial

Array Of Objects In Java How To Create Initialize And Use

Object Oriented Javascript For Beginners Learn Web Development Mdn
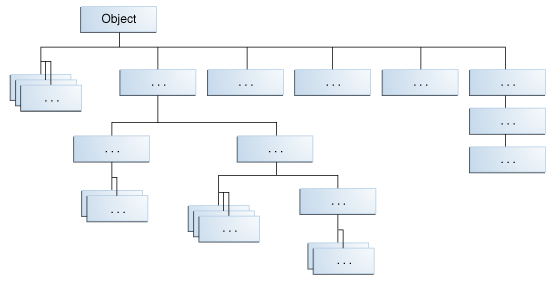
Inheritance The Java Tutorials Learning The Java Language Interfaces And Inheritance

Dependency Injection Using Spring Boot By Swatee Chand Edureka Medium

How Do I Instantiate An Object Of A Class Via Its String Name Web Tutorials Avajava Com
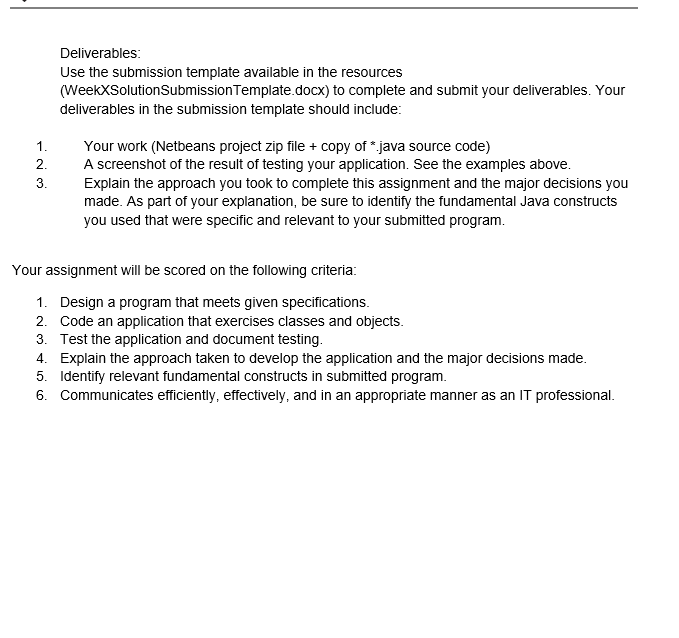
Solved Define Java Classes And Instantiate Their Objects Chegg Com
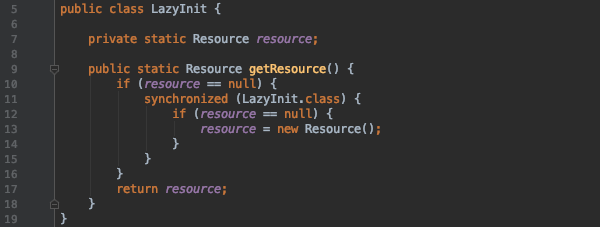
Lazy Initialisation What S A Correct Implementation By Maciej Najbar Androidpub

Factory Method Pattern Wikipedia
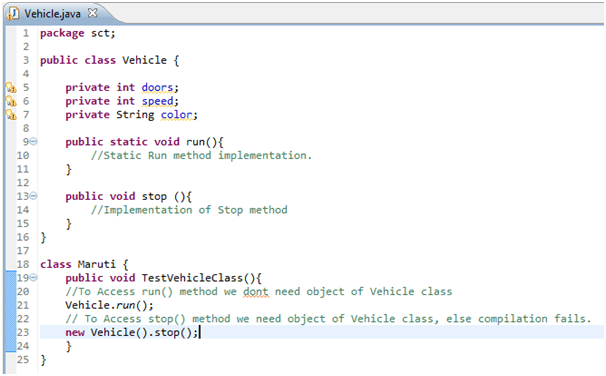
Java Class Methods Instance Variables W3resource

How To Initialize An Array In Java Journaldev
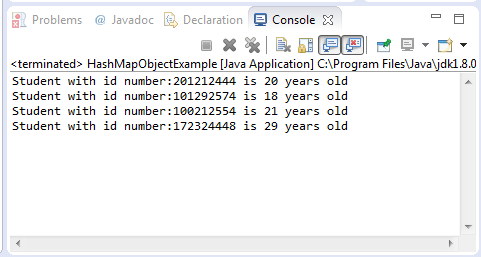
Java Hashmap Example
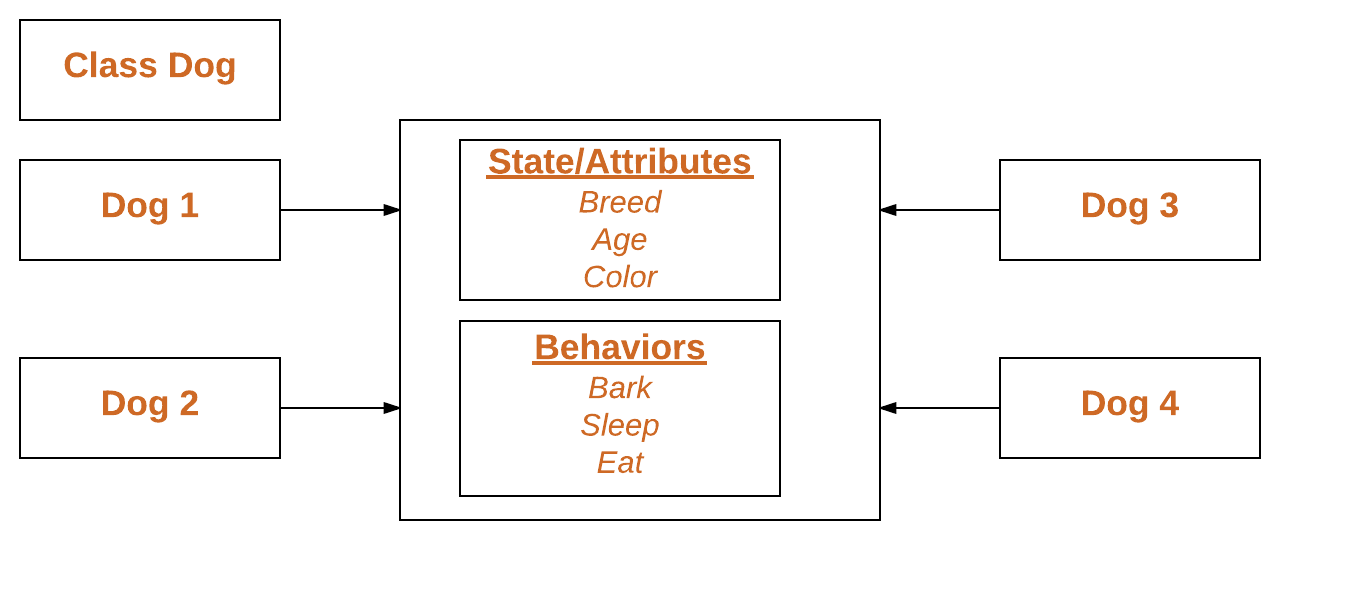
Classes And Objects In Java Geeksforgeeks

Fundamentals Of Java Static Method Class Variable And Block Crunchify
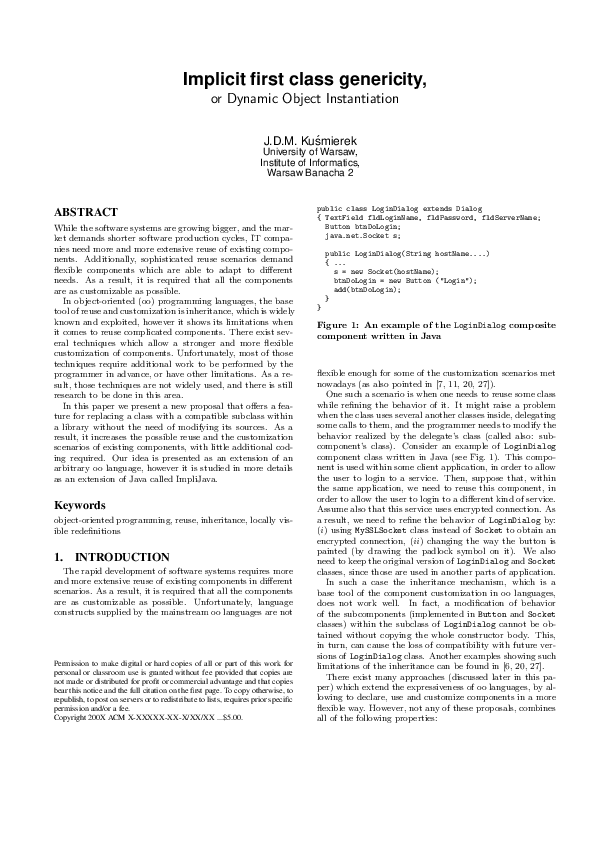
Pdf Implicit First Class Genericity Or Dynamic Object Instantiation Jarek Kusmierek Academia Edu
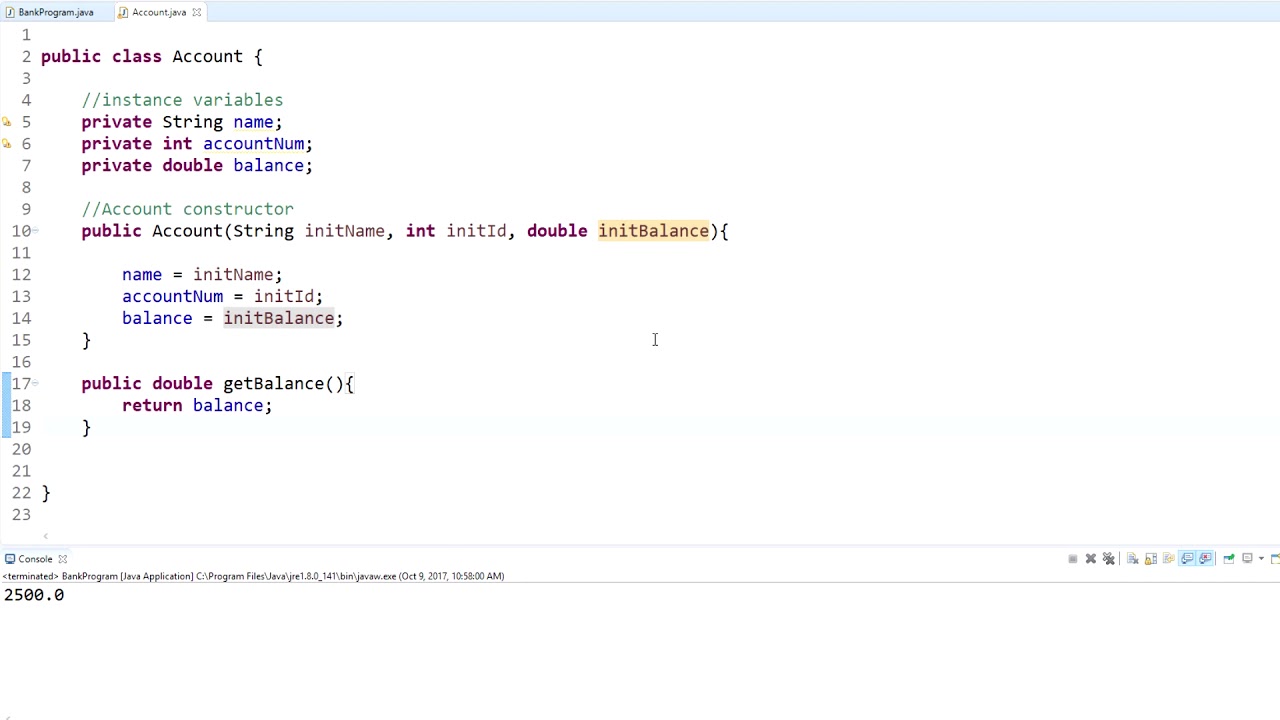
Java Programming Tutorial 15 Creating Instantiating Objects Youtube
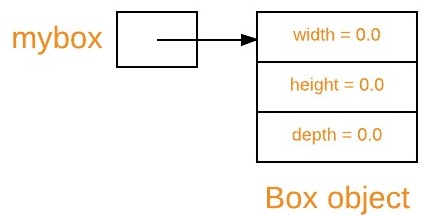
New Operator In Java Geeksforgeeks
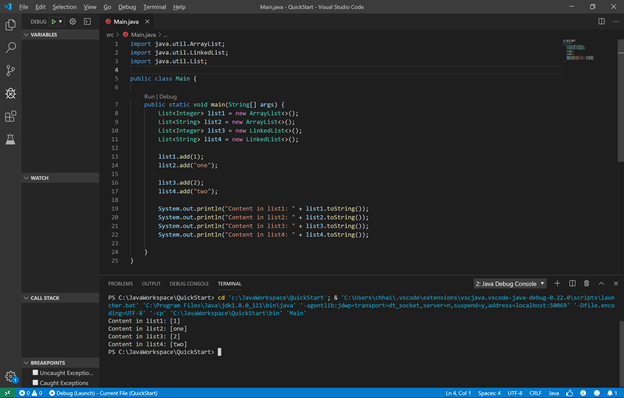
This Past Week I Learned Java Object Oriented Programming Polymorphism And Abstraction By Chhaian Pin Medium
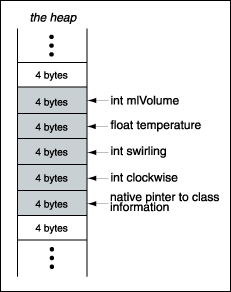
Object Initialization In Java
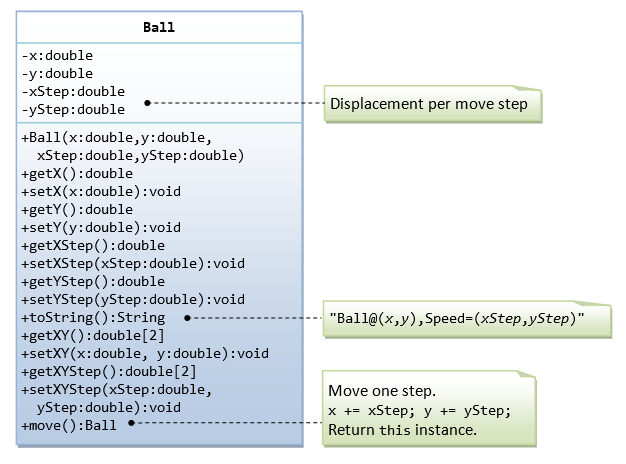
Oop Basics Java Programming Tutorial

Factory Method Pattern Wikipedia
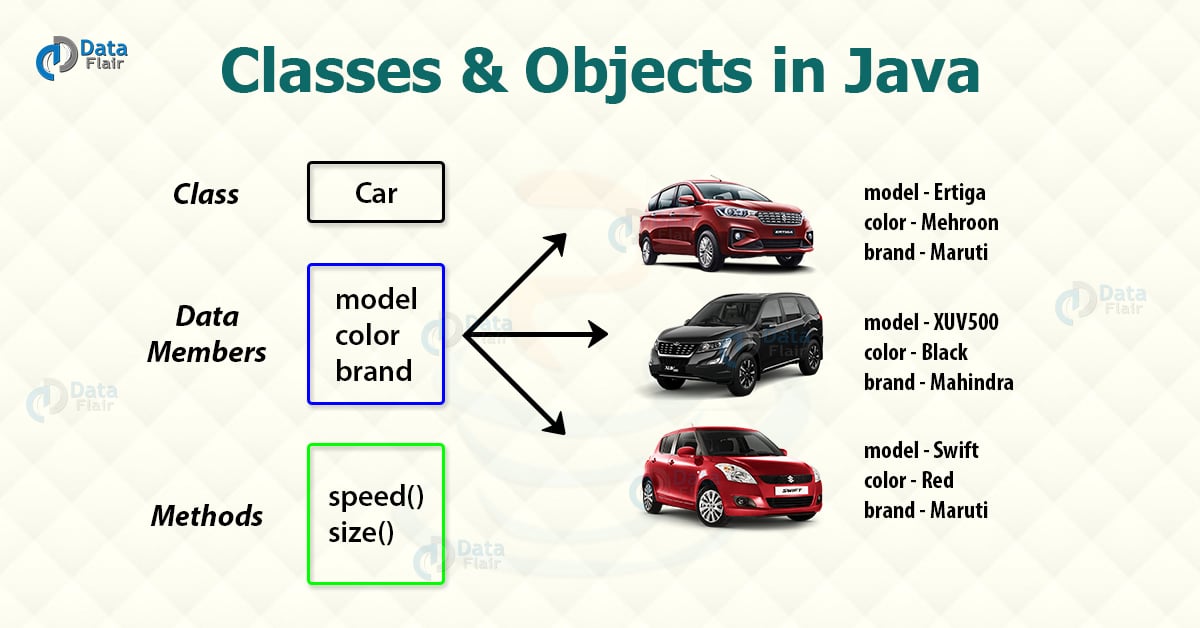
Classes And Objects In Java Fundamentals Of Oops Dataflair

Do I Need Constructors To Create An Object In Java Quora

Three Instantiation Methods Of Class Objects Programmer Sought

How To Instantiate An Object In Java Webucator

Java Fundamentals Tutorial Object Oriented Programming In Java

Thread Safe And A Fast Singleton Implementation Singleton Design Pattern In Java Crunchify
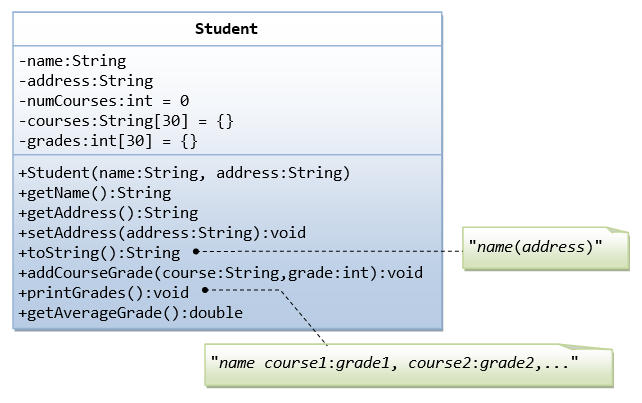
Oop Basics Java Programming Tutorial
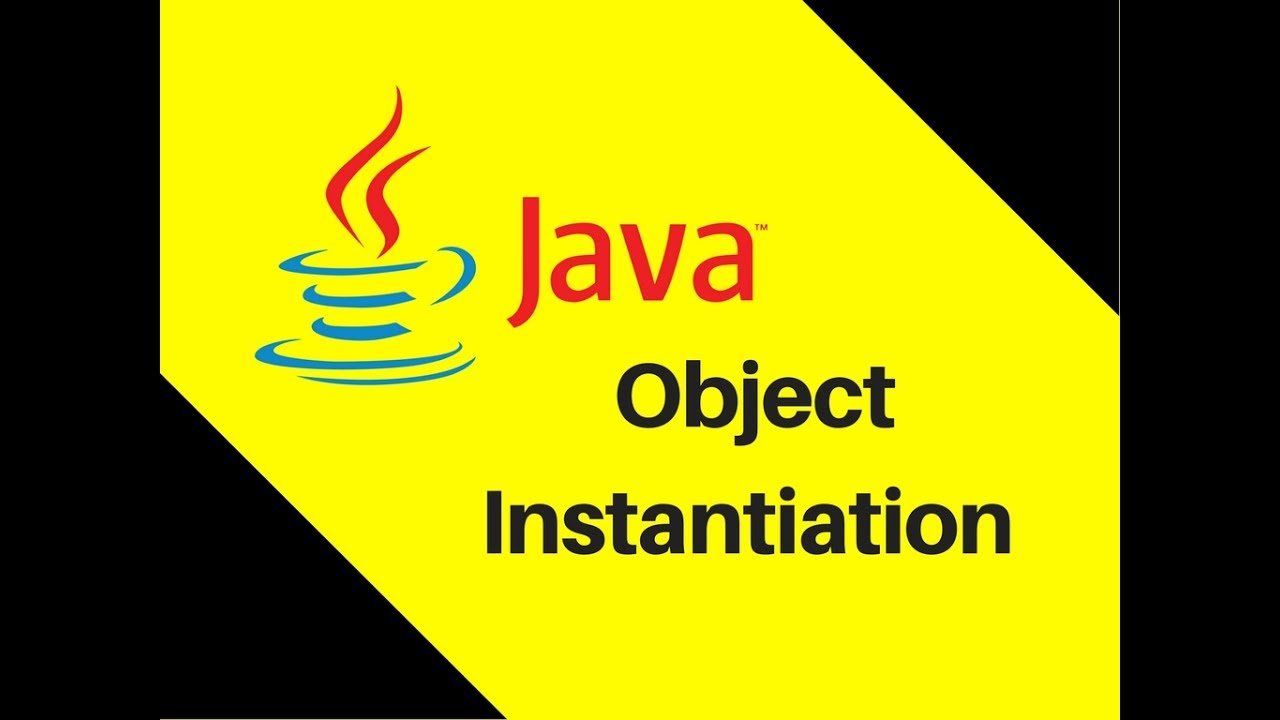
7 3 Object Instantiation How To Create Object In Java Youtube